Description
Computer Systems: A Programmer's Perspective, Global Edition (3rd Ed.)
Authors: Bryant Randal, O'Hallaron David
Language: English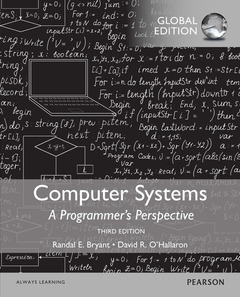
Subject for Computer Systems: A Programmer's Perspective, Global Edition:
95.64 €
In Print (Delivery period: 14 days).
Add to cart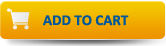
1120 p. · 18.6x24 cm · Paperback
Description
/li>Contents
/li>Comment
/li>
For courses in Computer Science and Programming
Computer systems: A Programmer?s Perspective explains the underlying elements common among all computer systems and how they affect general application performance. Written from the programmer?s perspective, this book strives to teach students how understanding basic elements of computer systems and executing real practice can lead them to create better programs.
Spanning across computer science themes such as hardware architecture, the operating system, and systems software, the 3rd Edition serves as a comprehensive introduction to programming. This book strives to create programmers who understand all elements of computer systems and will be able to engage in any application of the field--from fixing faulty software, to writing more capable programs, to avoiding common flaws. It lays the groundwork for students to delve into more intensive topics such as computer architecture, embedded systems, and cybersecurity.
This book focuses on systems that execute an x86-64 machine code, and recommends that students have access to a Linux system for this course. Students should have basic familiarity with C or C++.
- Part I: Program Structure and Execution
- Chapter 1: A Tour of Computer Systems
- Chapter 2: Representing and Manipulating Information
- Chapter 3: Machine-Level Representation of Programs
- Chapter 4: Processor Architecture
- Chapter 5: Optimizing Program Performance
- Chapter 6: The Memory Hierarchy
- Part II: Running Programs on a System
- Chapter 7: Linking
- Chapter 8: Exceptional Control Flow
- Chapter 9: Virtual Memory
- Part III: Interaction and Communication Between Programs
- Chapter 10: System-Level I/O
- Chapter 11: Network Programming
- Chapter 12: Concurrent Programming
- Appendix
- Error Handling
A carefully planned 12 chapter layout that covers all of the core topics of computer programming
- Chapter 1 uses a simple “hello world” program to introduce the major concepts and themes of computer programming.
- Chapter 2 dives into the topic of computer arithmetic, considering how numbers are represented in computer programs and how they affect value coding. The chapter places a special emphasis on the properties of unsigned and two’s-compliment number representations. It gives students necessary insight into arithmetic from the programmers perspective and why it’s so important.
- Chapter 3 teaches students how to read the x86-64 code generated by a C compiler, covering the basic instruction patterns for different control constructs, implementation procedures, and the allocation of different data structures. This chapter also discusses the implementation of integer and floating point arithmetic and takes a machine-level view of programs to understand certain code vulnerabilities.
- Chapter 4 introduces students to basic combinational and sequential logic elements and shows how they can be used in a simplified subset of the x86-64 instruction set called Y86-64. It starts with a single-cycle datapath and moves onto a discussion of pipelining.
- Chapter 5 gives students techniques for improving code performance with transformations that reduce work and enhance instruction-level parallelism.
- Chapter 6 covers different types of RAM and ROM memory systems, describing their hierarchical arrangement. The chapter makes the abstract concept tangible by using the analogy of a “memory mountain” with ridges of temporal locality and slopes of spatial locality. Students learn that improving temporal and spatial locality improves performance.
- Chapter 7 discusses both static and dynamic linkin