Description
Effective C# (Covers C# 6.0) (3rd Ed.)
50 Specific Ways to Improve Your C#
Effective Software Development Series
Author: Wagner Bill
Language: English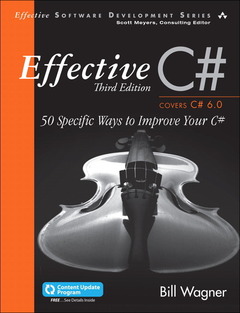
Subject for Effective C# (Covers C# 6.0):
288 p. · Paperback
Description
/li>Contents
/li>Biography
/li>Comment
/li>
In Effective C#, Third Edition, respected .NET expert Bill Wagner identifies 50 ways to harness the full power of the C# 6.0 language to write exceptionally robust, efficient, and well-performing code. Reflecting the growing sophistication of the C# language and its development community, Wagner has identified dozens of new ways to write better code. This edition?s new solutions include some that take advantage of generics and several that are more focused on LINQ, as well as a full chapter of advanced best practices for working with exceptions.
Wagner?s clear, practical explanations, expert tips, and realistic code examples have made Effective C# indispensable to hundreds of thousands of developers. Drawing on his unsurpassed C# experience, he addresses everything from resource management to multicore support, and reveals how to avoid common pitfalls in the language and its .NET environment. Learn how to choose the most effective solution when multiple options exist, and how to write code that?s far easier to maintain and improve. Wagner shows how and why to
- Prefer implicitly typed local variables (see Item 1)
- Replace string.Format() with interpolated strings (see Item 4)
- Express callbacks with delegates (see Item 7)
- Make the most of .NET resource management (see Item 11)
- Define minimal and sufficient constraints for generics (see Item 18)
- Specialize generic algorithms using runtime type checking (see Item 19)
- Use delegates to define method constraints on type parameters (see Item 23)
- Augment minimal interface contracts with extension methods (see Item 27)
- Create composable APIs for sequences (see Item 31)
- Decouple iterations from actions, predicates, and functions (see Item 32)
- Prefer lambda expressions to methods (see Item 38)
- Distinguish early from deferred execution (see Item 40)
- Avoid capturing expensive resources (see Item 41)
- Use exceptions to report method contract failures (see Item 45)
- Leverage side effects in exception filters (see Item 50)
You?re already a successful C# programmer, and this book will make you an outstanding one.
Content Update Program: This title is no longer part of the Content Update Program. The content is final, and no further updates will be released.
Introduction xiii
Chapter 1: C# Language Idioms 1
Item 1: Prefer Implicitly Typed Local Variables 1
Item 2: Prefer readonly to const 7
Item 3: Prefer the is or as Operators to Casts 12
Item 4: Replace string.Format() with Interpolated Strings 19
Item 5: Prefer FormattableString for Culture-Specific Strings 23
Item 6: Avoid String-ly Typed APIs 26
Item 7: Express Callbacks with Delegates 28
Item 8: Use the Null Conditional Operator for Event Invocations 31
Item 9: Minimize Boxing and Unboxing 34
Item 10: Use the new Modifier Only to React to Base Class Updates 38
Chapter 2: .NET Resource Management 43
Item 11: Understand .NET Resource Management 43
Item 12: Prefer Member Initializers to Assignment Statements 48
Item 13: Use Proper Initialization for Static Class Members 51
Item 14: Minimize Duplicate Initialization Logic 53
Item 15: Avoid Creating Unnecessary Objects 61
Item 16: Never Call Virtual Functions in Constructors 65
Item 17: Implement the Standard Dispose Pattern 68
Chapter 3: Working with Generics 77
Item 18: Always Define Constraints That Are Minimal and Sufficient 79
Item 19: Specialize Generic Algorithms Using Runtime Type Checking 85
Item 20: Implement Ordering Relations with IComparable<T> and IComparer<T> 92
Item 21: Always Create Generic Classes That Support Disposable Type Parameters 98
Item 22: Support Generic Covariance and Contravariance 101
Item 23: Use Delegates to Define Method Constraints on Type Parameters 107
Item 24: Do Not Create Generic Specialization on Base Classes or Interfaces 112
Item 25: Prefer Generic Methods Unless Type Parameters Are Instance Fields 116
Item 26: Implement Classic Interfaces in Addition to Generic Interfaces 120
Item 27: Augment Minimal Interface Contracts with Extension Methods 126
Item 28: Consider Enhancing Constructed Types with Extension Methods 130
Chapter 4: Working with LINQ 133
Item 29: Prefer Iterator Methods to Returning Collections 133
Item 30: Prefer Query Syntax to Loops 139
Item 31: Create Composable APIs for Sequences 144
Item 32: Decouple Iterations from Actions, Predicates, and Functions 151
Item 33: Generate Sequence Items as Requested 154
Item 34: Loosen Coupling by Using Function Parameters 157
Item 35: Never Overload Extension Methods 163
Item 36: Understand How Query Expressions Map to Method Calls 167
Item 37: Prefer Lazy Evaluation to Eager Evaluation in Queries 179
Item 38: Prefer Lambda Expressions to Methods 184
Item 39: Avoid Throwing Exceptions in Functions and Actions 188
Item 40: Distinguish Early from Deferred Execution 191
Item 41: Avoid Capturing Expensive Resources 195
Item 42: Distinguish between IEnumerable and IQueryable Data Sources 208
Item 43: Use Single() and First() to Enforce Semantic Expectations on Queries 212
Item 44: Avoid Modifying Bound Variables 215
Chapter 5: Exception Practices 221
Item 45: Use Exceptions to Report Method Contract Failures 221
Item 46: Utilize using and try/finally for Resource Cleanup 225
Item 47: Create Complete Application-Specific Exception Classes 232
Item 48: Prefer the Strong Exception Guarantee 237
Item 49: Prefer Exception Filters to catch and re-throw 245
Item 50: Leverage Side Effects in Exception Filters 249
Index 253
Bill Wagner creates .NET learning materials for Microsoft. He is one of the world’s foremost C# experts and is a member of the ECMA C# Standards Committee. The president of Humanitarian Toolbox, he also now serves on the .NET Foundation Advisory Council and Technical Steering Committees. Wagner has worked with companies ranging from start-ups to enterprises, improving development processes and growing development teams. An internationally recognized writer, he authored this book’s first two editions, as well as More Effective C#. He holds a B.S. in computer science from the University of Illinois at Champaign-Urbana.
Now fully updated for C# 6.0 and .NET 5.0: Bill Wagner's 50 high-performance techniques for fully harnessing C#'s power to write outstanding code!
- Up-to-the-minute C# practical advice, best practices, tips, shortcuts, and idioms it would take you years to discover on your own
- Go from good to great, fast! Helps experienced C# programmers gain a far deeper understanding, so they can make the most of the language
- Improve your code with Bill Wagner's exclusive Code Analyzer
- By Bill Wagner, world-renowned C# author, MVP, speaker, Microsoft programming insider, Regional Director, and ECMA standards committee member
- Follows the wildly-popular "Effective" format proven in Scott Meyers' classic Effective C++
These books may interest you
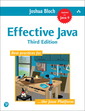
Effective Java 51.64 €