Description
Arduino Sketches
Tools and Techniques for Programming Wizardry
Author: Langbridge James A.
Language: English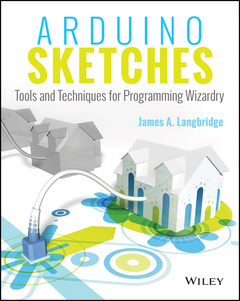
Subject for Arduino Sketches:
Keywords
Arduino Sketches; James A; Langbridge; beginner Arduino; Arduino programming; Arduino guide; Arduino help; Arduino tutorial; learn Arduino; Arduino examples; Arduino boards; Arduino language; advanced Arduino; Arduino LCD; Arduino Firmata; Arduino Due; Arduino Esplora; Arduino Yun; Arduino Robot; Arduino Xbees; Arduino libraries; Arduino TFT; Arduino Bridge; Arduino projects; Arduino communication; beginner robotics; Arduino workshop; Arduino cookbook; Arduino basic programming; Arduino fundamentals
480 p. · 18.8x23.4 cm · Paperback
Description
/li>Contents
/li>Biography
/li>
Arduino Sketches is a practical guide to programming the increasingly popular microcontroller that brings gadgets to life. Accessible to tech-lovers at any level, this book provides expert instruction on Arduino programming and hands-on practice to test your skills. You'll find coverage of the various Arduino boards, detailed explanations of each standard library, and guidance on creating libraries from scratch ? plus practical examples that demonstrate the everyday use of the skills you're learning. Work on increasingly advanced programming projects, and gain more control as you learn about hardware-specific libraries and how to build your own. Take full advantage of the Arduino API, and learn the tips and tricks that will broaden your skillset.
The Arduino development board comes with an embedded processor and sockets that allow you to quickly attach peripherals without tools or solders. It's easy to build, easy to program, and requires no specialized hardware. For the hobbyist, it's a dream come true ? especially as the popularity of this open-source project inspires even the major tech companies to develop compatible products. Arduino Sketches is a practical, comprehensive guide to getting the most out of your Arduino setup. You'll learn to:
- Communicate through Ethernet, WiFi, USB, Firmata, and Xbee
- Find, import, and update user libraries, and learn to create your own
- Master the Arduino Due, Esplora, Yun, and Robot boards for enhanced communication, signal-sending, and peripherals
- Play audio files, send keystrokes to a computer, control LED and cursor movement, and more
This book presents the Arduino fundamentals in a way that helps you apply future additions to the Arduino language, providing a great foundation in this rapidly-growing project. If you're looking to explore Arduino programming, Arduino Sketches is the toolbox you need to get started.
Introduction xxix
Part I Introduction to Arduino 1
Chapter 1 Introduction to Arduino 3
Atmel AVR 5
The Arduino Project 7
The ATmega Series 8
The ATmega Series 8
The ATtiny Series 8
Other Series 9
The Different Arduinos 9
Arduino Uno 10
Arduino Leonardo 10
Arduino Ethernet 11
Arduino Mega 2560 11
Arduino Mini 13
Arduino Micro 13
Arduino Due 13
LilyPad Arduino 14
Arduino Pro 16
Arduino Robot 16
Arduino Esplora 18
Arduino Yún 18
Arduino Tre 19
Arduino Zero 19
Your Own Arduino? 20
Shields 20
What Is a Shield? 20
The Different Shields 21
Arduino Motor Shield 21
Arduino Wireless SD Shield 21
Arduino Ethernet Shield 21
Arduino WiFi Shield 22
Arduino GSM Shield 22
Your Own Shield 22
What Can You Do with an Arduino? 22
What You Will Need for This Book 23
Summary 24
Chapter 2 Programming for the Arduino 25
Installing Your Environment 26
Downloading the Software 27
Running the Software 28
Using Your Own IDE 29
Your First Program 29
Understanding Your First Sketch 33
Programming Basics 36
Variables and Data Types 36
Control Structures 38
if Statement 38
switch Case 39
while Loop 40
for Loop 41
Functions 42
Libraries 42
Summary 42
Chapter 3 Electronics Basics 45
Electronics 101 46
Voltage, Amperage, and Resistance 46
Voltage 47
Amperage 48
Resistance 48
Ohm’s Law 49
The Basic Components 49
Resistors 50
Different Resistor Values 50
Identifying Resistor Values 50
Using Resistors 52
Capacitors 53
Using Capacitors 54
Diodes 54
Different Types of Diodes 54
Using Diodes 55
Light-Emitting Diodes 55
Using LEDs 55
Transistors 56
Using Transistors 56
Breadboards 56
Inputs and Outputs 57
Connecting a Light-Emitting Diode 58
Calculation 58
Software 59
Hardware 60
What Now? 61
Summary 61
Part II Standard Libraries 63
Chapter 4 The Arduino Language 65
I/O Functions 65
Digital I/O 65
pinMode() 66
digitalRead() 66
digitalWrite() 67
Analog I/O 67
analogRead() 68
analogWrite() 68
Generating Audio Tones 69
tone() 69
noTone() 69
Reading Pulses 69
pulseIn() 70
Time Functions 70
delay() 70
delayMicroseconds() 71
millis() 71
micros() 71
Mathematical Functions 72
min() 72
max() 72
constrain() 73
abs() 73
map() 73
pow() 74
sqrt() 74
random() 74
Trigonometry 75
sin() 76
cos() 76
tan() 76
Constants 76
Interrupts 76
attachInterrupt() 77
detachInterrupt() 78
noInterrupts() 78
interrupts() 78
Summary 79
Chapter 5 Serial Communication 81
Introducing Serial Communication 82
UART Communications 84
Baud Rate 84
Data Bits 85
Parity 85
Stop Bits 86
Debugging and Output 86
Starting a Serial Connection 87
Writing Data 88
Sending Text 88
Sending Data 90
Reading Data 91
Starting Communications 91
Is Data Waiting? 91
Reading a Byte 92
Reading Multiple Bytes 92
Taking a Peek 93
Parsing Data 93
Cleaning Up 94
Example Program 95
SoftwareSerial 98
Summary 99
Chapter 6 EEPROM 101
Introducing EEPROM 101
The Different Memories on Arduino 103
The EEPROM Library 104
Reading and Writing Bytes 104
Reading and Writing Bits 105
Reading and Writing Strings 107
Reading and Writing Other Values 108
Example Program 110
Preparing EEPROM Storage 113
Adding Nonvolatile Memory 114
Summary 115
Chapter 7 SPI 117
Introducting SPI 118
SPI Bus 118
Comparison to RS-232 119
Confi guration 119
Communications 120
Arduino SPI 120
SPI Library 121
SPI on the Arduino Due 123
Example Program 125
Hardware 126
Sketch 128
Exercises 131
Summary 132
Chapter 8 Wire 133
Introducing Wire 134
Connecting I2C 135
I2C Protocol 135
Address 136
Communication 137
Communicating 138
Master Communications 139
Sending Information 139
Requesting Information 140
Slave Communications 141
Receiving Information 141
Sending Information 142
Example Program 142
Exercises 146
Traps and Pitfalls 147
Voltage Difference 147
Bus Speed 147
Shields with I2C 148
Summary 148
Chapter 9 Ethernet 149
Introduction 149
Ethernet 150
Ethernet Cables 151
Switches and Hubs 151
PoE 152
TCP/IP 152
MAC Address 153
IP Address 153
DNS 153
Port 153
Ethernet on Arduino 154
Importing the Ethernet Library 154
Starting Ethernet 155
Arduino as a Client 157
Sending and Receiving Data 158
Connecting to a Web Server 159
Example Program 161
Arduino as a Server 163
Serving Web Pages 164
Example Program 165
Sketch 165
Summary 167
Chapter 10 WiFi 169
Introduction 170
The WiFi Protocol 171
Topology 171
Network Parameters 172
Channels 172
Encryption 172
SSID 173
RSSI 173
Arduino WiFi 173
Importing the Library 174
Initialization 174
Status 175
Scanning Networks 176
Connecting and Configuring 177
Wireless Client 178
Wireless Server 179
Example Application 179
Hardware 181
Sketch 182
Exercises 189
Summary 190
Chapter 11 LiquidCrystal 191
Introduction 192
LiquidCrystal Library 194
Writing Text 195
Cursor Commands 196
Text Orientation 197
Scrolling 197
Custom Text 198
Example Program 199
Hardware 200
Software 201
Exercises 205
Summary 205
Chapter 12 SD 207
Introduction 208
SD Cards 211
Capacity 212
Speed 213
Using SD Cards with Arduino 213
Accepted SD Cards 214
Limitations 214
The SD Library 215
Importing the Library 215
Connecting a Card 215
Opening and Closing Files 216
Reading and Writing Files 217
Reading Files 217
Writing Files 218
Folder Operations 218
Card Operations 219
Advanced Usage 220
Example Program and Sketch 220
Summary 224
Chapter 13 TFT 225
Introduction 226
Technologies 227
TFT Library 228
Initialization 228
Screen Preparation 229
Text Operations 230
Basic Graphics 231
Coloring 232
Graphic Images 232
Example Application 233
Hardware 234
Sketch 234
Exercises 239
Summary 239
Chapter 14 Servo 241
Introduction to Servo Motors 242
Controlling Servo Motors 243
Connecting a Servo Motor 243
Moving Servo Motors 244
Disconnecting 245
Precision and Safety 246
Example Application 246
Schematic 248
Sketch 249
Exercises 250
Summary 251
Chapter 15 Stepper 253
Introducing Motors 254
Controlling a Stepper Motor 254
Hardware 255
Unipolar Versus Bipolar Stepper Motors 255
The Stepper Library 256
Example Project 257
Hardware 257
Sketch 258
Summary 260
Chapter 16 Firmata 261
Introducing Firmata 262
Firmata Library 262
Sending Messages 263
Receiving Messages 263
Callbacks 264
SysEx 266
Example Program 268
Summary 269
Chapter 17 GSM 271
Introducing GSM 272
Mobile Data Network 272
GSM 273
GPRS 274
EDGE 274
3 G 274
4 G and the Future 275
Modems 275
Arduino and GSM 276
Arduino GSM Library 276
GSM Class 278
SMS Class 279
VoiceCall Class 281
GPRS 282
Modem 284
Example Application 285
Summary 288
Part III Device-Specific Libraries 289
Chapter 18 Audio 291
Introducing Audio 292
Digital Sound Files 292
Music on the Arduino 294
Arduino Due 294
Digital to Analog Converters 295
Digital Audio to Analog 295
Creating Digital Audio 296
Storing Digital Audio 296
Playing Digital Audio 296
Example Program 298
Hardware 298
Sketch 300
Exercise 303
Summary 304
Chapter 19 Scheduler 305
Introducing Scheduling 306
Arduino Multitasking 307
Scheduler 308
Cooperative Multitasking 309
Noncooperative Functions 311
Example Program 313
Hardware 314
Sketch 316
Exercises 319
Summary 319
Chapter 20 USBHost 321
Introducing USBHost 322
USB Protocol 323
USB Devices 324
Keyboards 324
Mice 325
Hubs 325
Arduino Due 325
USBHost Library 327
Keyboards 327
Mice 329
Example Program 330
Hardware 331
Source Code 332
Summary 334
Chapter 21 Esplora 335
Introducing Esplora 336
The Arduino Esplora Library 337
RGB LED 337
Sensors 338
Buttons 339
Buzzer 340
TinkerKit 341
LCD Module 342
Example Program and Exercises 342
Summary 344
Chapter 22 Robot 345
Introducing Robot Library 346
Arduino Robot 348
Robot Library 349
Control Board 350
Robotic Controls 350
Sensor Reading 351
Personalizing Your Robot 353
LCD Screen 354
Music 356
Motor Board 357
Example Program and Exercises 358
Summary 360
Chapter 23 Bridge 361
Introducing Bridge Library 362
Bridge 363
Process 364
FileIO 366
YunServer 367
YunClient 368
Example Application 369
Hardware 369
Sketch 370
Exercises 373
Summary 373
Part IV User Libraries and Shields 375
Chapter 24 Importing Third-Party Libraries 377
Libraries 378
Finding Libraries 378
Importing a Library 379
Using an External Library 381
Example Application 384
Exercises 389
Summary 389
Chapter 25 Creating Your Own Shield 391
Creating a Shield 391
The Idea 392
The Required Hardware 392
The Required Software 393
Your First Shield 394
Step 1: The Breadboard 395
Step 2: The Schematic 398
Step 3: The PCB 402
Summary 404
Chapter 26 Creating Your Own Library 405
Libraries 405
Library Basics 406
Simple Libraries 406
Advanced Libraries 410
Adding Comments 413
Adding Examples 415
Read Me 415
Coding Style 416
Use CamelCase 416
Use English Words 416
Don’t Use External Libraries 417
Use Standard Names 417
Distributing Your Library 417
Closed Source Libraries 417
Example Library 418
The Library 418
Examples 424
README 427
Finishing Touches 428
Summary 428
Index 429
James A. Langbridge is a software consultant specializing in embedded systems and code optimization including bootl oader code, system initialization, and code optimization. He has a history in in robotics, mobile phones, and seismic detection systems, with over 10 years in the aviation, defense, industry and telecom sectors, and served as an R&D consultant for ST Microelectronics and Amtel. James has also been called upon to train development teams and coach junior developers to their maximum potential.
These books may interest you
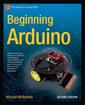
Beginning Arduino 68.56 €