Description
Ivor horton's beginning visual c++ 2012 (paperback)
Author: Horton Ivor
Language: English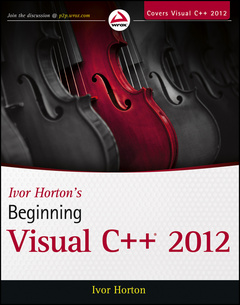
Subject for Ivor horton's beginning visual c++ 2012 (paperback):
Keywords
Visual C++; Visual C++ 2012; learning Visual C++; books on Visual C++; books on C++11; tutorials on Visual C++; programming tutorials; beginning programming books; beginning books on Visual C++; learn to use Visual C++ 2012; Ivor Horton; books by Ivor Horton; beginning Visual C++; C++11; C++11 and Visual Studio 2012; instructions on C++11 and Visual Studio 2012; books on visual studio; how to program in visual c++; c++; about c++11; about c++11; programming guides; c++ programming guides
Approximative price 66.44 €
In Print (Delivery period: 12 days).
Add to cart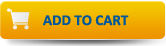
984 p. · 18.8x23.6 cm · Paperback
Description
/li>Contents
/li>Biography
/li>
There's a reason why Ivor Horton's Beginning Visual C++ books dominate the marketplace. Ivor Horton has a loyal following who love his winning approach to teaching programming languages, and in this fully updated new edition, he repeats his successful formula. Offering a comprehensive introduction to both the standard C++ language and to Visual C++, he offers step-by-step programming exercises, examples, and solutions to deftly guide novice programmers through the ins and outs of C++ development.
- Introduces novice programmers to the current standard, Microsoft Visual C++ 2012, as it is implemented in Microsoft Visual Studio 2012
- Focuses on teaching both the C++11 standard and Visual C++ 2012, unlike virtually any other book on the market
- Covers the C++ language and library and the IDE
- Delves into new features of both the C++11 standard and of the Visual C++ 2012 programming environment
- Features C++ project templates, code snippets, and more
Even if you have no previous programming experience, you'll soon learn how to build real-world applications using Visual C++ 2012 with this popular guide.
CHAPTER 1: PROGRAMMING WITH VISUAL C++ 1
Learning with Visual C++ 1
Writing C++ Applications 2
Learning Desktop Applications Programming 3
Learning C++ 3
Console Applications 4
Windows Programming Concepts 4
What Is the Integrated Development Environment? 5
The Editor 6
The Compiler 6
The Linker 6
The Libraries 6
Using the IDE 7
Toolbar Options 8
Dockable Toolbars 9
Documentation 10
Projects and Solutions 10
Defining a Project 10
Debug and Release Versions of Your Program 15
Executing the Program 16
Dealing with Errors 18
Setting Options in Visual C++ 19
Creating and Executing Windows Applications 20
Creating an MFC Application 20
Building and Executing the MFC Application 22
Summary 23
CHAPTER 2: DATA, VARIABLES, AND CALCULATIONS 25
The Structure of a C++ Program 26
Program Comments 31
The #include Directive — Header Files 32
Namespaces and the Using Declaration 33
The main() Function 34
Program Statements 34
Whitespace 37
Statement Blocks 37
Automatically Generated Console Programs 37
Precompiled Header Files 38
Main Function Names 39
Defining Variables 39
Naming Variables 39
Keywords in C++ 40
Declaring Variables 41
Initial Values for Variables 41
Fundamental Data Types 42
Integer Variables 42
Character Data Types 44
Integer Type Modifi ers 45
The Boolean Type 46
Floating-Point Types 47
Fundamental Types in C++ 47
Literals 48
Defining Synonyms for Data Types 49
Basic Input/Output Operations 50
Input from the Keyboard 50
Output to the Command Line 50
Formatting the Output 51
Escape Sequences 53
Calculating in C++ 55
The Assignment Statement 55
Arithmetic Operations 55
The const Modifi er 58
Constant Expressions 58
Program Input 59
Calculating the Result 59
Displaying the Result 60
Calculating a Remainder 61
Modifying a Variable 61
The Increment and Decrement Operators 62
The Sequence of Calculation 65
Operator Precedence 65
Type Conversion and Casting 66
Type Conversion in Assignments 67
Explicit Type Conversion 68
Old-Style Casts 69
The auto Keyword 69
Discovering Types 70
The Bitwise Operators 70
The Bitwise AND 71
The Bitwise OR 72
The Bitwise Exclusive OR 73
The Bitwise NOT 74
The Bitwise Shift Operators 74
Introducing Lvalues and Rvalues 76
Understanding Storage Duration and Scope 77
Automatic Variables 78
Positioning Variable Declarations 80
Global Variables 80
Static Variables 84
Variables with Specifi c Sets of Values 85
Old Enumerations 85
Type-Safe Enumerations 87
Namespaces 90
Declaring a Namespace 91
Multiple Namespaces 92
Summary 93
CHAPTER 3: DECISIONS AND LOOPS 97
Comparing Values 97
The if Statement 99
Nested if Statements 100
The Extended if Statement 102
Nested if-else Statements 104
Logical Operators and Expressions 106
Logical AND 107
Logical OR 107
Logical NOT 108
The Conditional Operator 109
The switch Statement 111
Unconditional Branching 115
Repeating a Block of Statements 115
What Is a Loop? 116
Variations on the for Loop 118
Using the continue Statement 122
Floating-Point Loop Counters 126
The while Loop 126
The do-while Loop 128
The Range-Based for Loop 129
Nested Loops 130
Summary 133
CHAPTER 4: ARRAYS, STRINGS, AND POINTERS 135
Handling Multiple Data Values
of the Same Type 135
Arrays 136
Declaring Arrays 137
Initializing Arrays 140
Using the Range-Based for Loop 142
Character Arrays and String Handling 143
String Input 144
Using the Range-Based for Loop with Strings 146
Multidimensional Arrays 146
Initializing Multidimensional Arrays 147
Indirect Data Access 150
What Is a Pointer? 150
Declaring Pointers 150
The Address-Of Operator 151
Using Pointers 152
The Indirection Operator 152
Why Use Pointers? 152
Initializing Pointers 152
Pointers to char 155
The sizeof Operator 159
Constant Pointers and Pointers to Constants 161
Pointers and Arrays 163
Pointer Arithmetic 164
Using Pointers with Multidimensional Arrays 168
Pointer Notation with Multidimensional Arrays 169
Dynamic Memory Allocation 170
The Free Store, Alias the Heap 170
The new and delete Operators 171
Allocating Memory Dynamically for Arrays 172
Dynamic Allocation of Multidimensional Arrays 175
Using References 176
What Is a Reference? 176
Declaring and Initializing Lvalue References 176
Using References in a Range-Based for Loop 177
Rvalue References 178
Library Functions for Strings 178
Finding the Length of a Null-Terminated String 179
Joining Null-Terminated Strings 179
Copying Null-Terminated Strings 181
Comparing Null-Terminated Strings 182
Searching Null-Terminated Strings 183
Summary 185
CHAPTER 5: INTRODUCING STRUCTURE
INTO YOUR PROGRAMS 189
Understanding Functions 189
Why Do You Need Functions? 191
Structure of a Function 191
The Function Header 191
The Function Body 193
The return Statement 193
Alternative Function Syntax 194
Using a Function 194
Function Prototypes 194
Passing Arguments to a Function 198
The Pass-by-Value Mechanism 199
Pointers as Arguments to a Function 200
Passing Arrays to a Function 202
Passing Multidimensional Arrays to a Function 204
References as Arguments to a Function 206
Use of the const Modifi er 208
Rvalue Reference Parameters 210
Arguments to main( ) 212
Accepting a Variable Number of Function Arguments 214
Returning Values from a Function 216
Returning a Pointer 216
A Cast-Iron Rule for Returning Addresses 218
Returning a Reference 219
A Tefl on-Coated Rule: Returning References 222
Static Variables in a Function 222
Recursive Function Calls 224
Using Recursion 227
Summary 227
CHAPTER 6: MORE ABOUT PROGRAM STRUCTURE 231
Pointers to Functions 231
Declaring Pointers to Functions 232
A Pointer to a Function as an Argument 235
Arrays of Pointers to Functions 237
Initializing Function Parameters 238
Exceptions 239
Throwing Exceptions 241
Catching Exceptions 242
Rethrowing Exceptions 244
Exception Handling in the MFC 244
Handling Memory Allocation Errors 245
Function Overloading 247
What Is Function Overloading? 247
Reference Types and Overload Selection 250
When to Overload Functions 251
Function Templates 251
Using a Function Template 251
Using the decltype Operator 254
An Example Using Functions 256
Implementing a Calculator 257
Analyzing the Problem 257
Eliminating Blanks from a String 260
How the Function Functions 260
Evaluating an Expression 260
How the Function Functions 262
Getting the Value of a Term 263
How the Function Functions 264
Analyzing a Number 264
How the Function Functions 266
Putting the Program Together 268
How the Function Functions 269
Extending the Program 269
How the Function Functions 271
Extracting a Substring 271
How the Function Functions 273
Running the Modified Program 273
Summary 274
CHAPTER 7: DEFINING YOUR OWN DATA TYPES 277
The struct in C++ 277
What Is a struct? 278
Defining a struct 278
Initializing a struct 279
Accessing the Members of a struct 279
IntelliSense Assistance with Structures 283
The struct RECT 285
Using Pointers with a struct 285
Accessing Structure Members through a Pointer 286
The Indirect Member Selection Operator 287
Types, Objects, Classes, and Instances 287
First Class 289
Operations on Classes 289
Terminology 290
Understanding Classes 290
Defining a Class 291
Access Control in a Class 291
Declaring Objects of a Class 291
Accessing the Data Members of a Class 292
Member Functions of a Class 294
Positioning a Member Function Defi nition 296
Inline Functions 297
Class Constructors 298
What Is a Constructor? 298
The Default Constructor 300
Default Parameter Values 303
Using an Initialization List in a Constructor 305
Making a Constructor Explicit 306
Private Members of a Class 307
Accessing private Class Members 310
The friend Functions of a Class 310
Placing friend Function Definitions Inside the Class 312
The Default Copy Constructor 313
The Pointer this 314
const Objects 317
const Member Functions of a Class 318
Member Function Definitions Outside the Class 319
Arrays of Objects 320
Static Members of a Class 322
Static Data Members 322
Static Function Members of a Class 325
Pointers and References to Objects 325
Pointers to Objects 326
References to Class Objects 328
Implementing a Copy Constructor 329
Summary 330
CHAPTER 8: MORE ON CLASSES 333
Class Destructors 334
What Is a Destructor? 334
The Default Destructor 334
Destructors and Dynamic Memory Allocation 337
Implementing a Copy Constructor 340
Sharing Memory Between Variables 342
Defining Unions 343
Anonymous Unions 344
Unions in Classes and Structures 345
Operator Overloading 345
Implementing an Overloaded Operator 346
Implementing Full Support for Comparison Operators 350
Overloading the Assignment Operator 354
Fixing the Problem 355
Overloading the Addition Operator 359
Overloading the Increment and Decrement Operators 364
Overloading the Function Call Operator 365
The Object Copying Problem 366
Avoiding Unnecessary Copy Operations 367
Applying Rvalue Reference Parameters 370
Named Objects are Lvalues 372
Default Class Members 377
Class Templates 378
Defining a Class Template 379
Template Member Functions 381
Creating Objects from a Class Template 382
Class Templates with Multiple Parameters 386
Templates for Function Objects 388
Perfect Forwarding 388
Using Classes 392
The Idea of a Class Interface 392
Defining the Problem 393
Implementing the CBox Class 393
Comparing CBox Objects 395
Combining CBox Objects 396
Analyzing CBox Objects 398
Organizing Your Program Code 412
Naming Program Files 413
Library Classes for Strings 414
Creating String Objects 414
Concatenating Strings 416
Accessing and Modifying Strings 420
Comparing Strings 424
Searching Strings 428
Summary 437
CHAPTER 9: CLASS INHERITANCE AND VIRTUAL FUNCTIONS 441
Object-Oriented Programming Basics 441
Inheritance in Classes 443
What Is a Base Class? 443
Deriving Classes from a Base Class 444
Access Control Under Inheritance 447
Constructor Operation in a Derived Class 450
Declaring Protected Class Members 454
The Access Level of Inherited Class Members 457
The Copy Constructor in a Derived Class 458
Preventing Class Derivation 461
Class Members as Friends 462
Friend Classes 464
Limitations on Class Friendship 464
Virtual Functions 464
What Is a Virtual Function? 467
Ensuring Correct Virtual Function Operation 469
Preventing Function Overriding 469
Using Pointers to Class Objects 470
Using References with Virtual Functions 472
Incomplete Class Declaration 473
Pure Virtual Functions 473
Abstract Classes 474
Indirect Base Classes 477
Virtual Destructors 479
Casting Between Class Types 483
Nested Classes 483
Summary 487
CHAPTER 10: THE STANDARD TEMPLATE LIBRARY 491
What Is the Standard Template Library? 491
Containers 492
Allocators 494
Comparators 494
Container Adapters 495
Iterators 495
Iterator Categories 495
SCARY Iterators 497
std::begin( ) and std::end( ) Functions 497
Smart Pointers 497
Using unique_ptr Objects 498
Using shared_ptr Objects 499
Accessing the Raw Pointer in a Smart Pointer 500
Casting SmartPointers 500
Algorithms 500
Function Objects in the STL 501
Function Adapters 502
The Range of STL Containers 502
Sequence Containers 502
Creating Vector Containers 504
The Capacity and Size of a Vector Container 507
Accessing the Elements in a Vector 512
Inserting and Deleting Elements in a Vector 513
Insert Operations 513
Emplace Operations 514
Erase Operations 515
Swap and Assign Operations 515
Storing Class Objects in a Vector 516
Sorting Vector Elements 522
Storing Pointers in a Vector 523
Array Containers 526
Double-Ended Queue Containers 529
Using List Containers 533
Adding Elements to a List 533
Accessing Elements in a List 535
Sorting List Elements 535
Other Operations on Lists 538
Using forward_list Containers 544
Using Other Sequence Containers 546
Queue Containers 546
Priority Queue Containers 549
Stack Containers 555
The tuple Class Template 557
Associative Containers 561
Using Map Containers 561
Storing Objects 562
Accessing Objects 564
Other Map Operations 565
Using a Multimap Container 574
More on Iterators 575
Using Input Stream Iterators 575
Using Inserter Iterators 578
Using Output Stream Iterators 580
More on Function Objects 582
More on Algorithms 584
Type Traits and Static Assertions 587
Lambda Expressions 588
The Capture Clause 589
Capturing Specifi c Variables 590
Templates and Lambda Expressions 591
Naming a Lambda Expression 595
Summary 598
CHAPTER 11: WINDOWS PROGRAMMING CONCEPTS 601
Windows Programming Basics 602
Elements of a Window 602
Windows Programs and the Operating System 604
Event-Driven Programs 605
Windows Messages 605
The Windows API 605
Windows Data Types 606
Notation in Windows Programs 607
The Structure of a Windows Program 608
The WinMain( ) Function 609
Specifying a Program Window 611
Creating a Program Window 613
Initializing the Program Window 615
Dealing with Windows Messages 616
A Complete WinMain( ) Function 620
How It Works 621
Processing Windows Messages 621
The WindowProc( ) Function 622
Decoding a Windows Message 622
Ending the Program 625
A Complete WindowProc( ) Function 625
How It Works 626
The Microsoft Foundation Classes 627
MFC Notation 627
How an MFC Program Is Structured 628
Summary 632
CHAPTER 12: WINDOWS PROGRAMMING
WITH THE MICROSOFT FOUNDATION
CLASSES (MFC) 635
The MFC Document/View Concept 636
What Is a Document? 636
Document Interfaces 636
What Is a View? 636
Linking a Document and Its Views 637
Document Templates 638
Document Template Classes 638
Your Application and MFC 639
Creating MFC Applications 640
Creating an SDI Application 641
MFC Application Wizard Output 645
Viewing Project Files 647
Viewing Classes 647
The Class Definitions 648
Creating an Executable Module 653
Running the Program 653
How the Program Works 654
Creating an MDI Application 655
Running the Program 656
Summary 657
CHAPTER 13: WORKING WITH MENUS AND TOOLBARS 659
Communicating with Windows 659
Understanding Message Maps 660
Message Handler Definitions 661
Message Categories 662
Handling Messages in Your Program 663
How Command Messages Are Processed 664
Extending the Sketcher Program 664
Elements of a Menu 665
Creating and Editing Menu Resources 665
Adding a Menu Item to the Menu Bar 666
Adding Items to the Element Menu 667
Modifying Existing Menu Items 667
Completing the Menu 668
Adding Menu Message Handlers 668
Choosing a Class to Handle Menu Messages 670
Creating Menu Message Handlers 670
Implementing Menu Message Handlers 672
Adding Members to Store Color and Element Mode 672
Defining Element and Color Types 674
Initializing the Color and Element Type Members 675
Implementing Menu Command Message Handlers 675
Running the Extended Example 676
Adding Menu Update Message Handlers 676
Coding a Command Update Handler 677
Exercising the Update Handlers 678
Adding Toolbar Buttons 678
Editing Toolbar Button Properties 680
Exercising the Toolbar Buttons 681
Adding Tooltips 682
Summary 682
CHAPTER 14: DRAWING IN A WINDOW 685
Basics of Drawing in a Window 685
The Window Client Area 686
The Windows Graphical Device Interface 686
Working with a Device Context 687
Mapping Modes 687
The MFC Drawing Mechanism 689
The View Class in Your Application 689
The OnDraw( ) Member Function 690
The CDC Class 691
Displaying Graphics 691
Drawing in Color 695
Drawing Graphics in Practice 700
Programming for the Mouse 702
Messages from the Mouse 703
WM_LBUTTONDOWN 704
WM_MOUSEMOVE 704
WM_LBUTTONUP 704
Mouse Message Handlers 704
Drawing Using the Mouse 706
Getting the Client Area Redrawn 708
Defining Element Classes 709
The CElement Class 713
The CLine Class 714
The CRectangle Class 717
The CCircle Class 719
The CCurve Class 722
Completing the Mouse Message Handlers 724
Drawing a Sketch 731
Running the Example 732
Capturing Mouse Messages 733
Summary 734
CHAPTER 15: IMPROVING THE VIEW 739
Sketcher Limitations 739
Improving the View 740
Updating Multiple Views 740
Scrolling Views 742
Logical Coordinates and Client Coordinates 744
Dealing with Client Coordinates 745
Using MM_LOENGLISH Mapping Mode 747
Deleting and Moving Elements 748
Implementing a Context Menu 748
Associating a Menu with a Class 750
Checking Context Menu Items 751
Identifying an Element under the Cursor 752
Exercising the Context Menus 754
Highlighting Elements 754
Drawing Highlighted Elements 757
Exercising the Highlights 757
Implementing Move and Delete 758
Deleting an Element 758
Moving an Element 758
Updating Other Views 761
Getting the Elements to Move Themselves 762
Dropping the Element 764
Exercising the Application 765
Dealing with Masked Elements 765
Summary 767
CHAPTER 16: WORKING WITH DIALOGS
AND CONTROLS 769
Understanding Dialogs 770
Understanding Controls 770
Creating a Dialog Resource 771
Adding Controls to a Dialog 771
Testing the Dialog 773
Programming for a Dialog 773
Adding a Dialog Class 773
Modal and Modeless Dialogs 774
Displaying a Dialog 775
Displaying the Dialog 776
Code to Close the Dialog 776
Supporting the Dialog Controls 777
Initializing Dialog Controls 778
Handling Radio Button Messages 779
Completing Dialog Operations 780
Adding Pen Widths to the Document 780
Adding Pen Widths to the Elements 781
Creating Elements in the View 783
Exercising the Dialog 784
Using a Spin Button Control 785
Adding a Scale Menu Item and Toolbar Button 785
Creating the Spin Button 786
The Controls’ Tab Sequence 786
Generating the Scale Dialog Class 787
Dialog Data Exchange and Validation 788
Initializing the Dialog 789
Displaying the Spin Button 790
Using the Scale Factor 791
Scalable Mapping Modes 791
Setting the Document Size 793
Setting the Mapping Mode 793
Implementing Scrolling with Scaling 795
Setting Up the Scrollbars 796
Working with Status Bars 797
Adding a Status Bar to a Frame 797
Creating Status Bar Panes 798
Updating the Status Bar 800
The CString Class 801
Using an Edit Box Control 802
Creating an Edit Box Resource 803
Creating the Dialog Class 804
Adding the Text Menu Item 805
Defining a Text Element 806
Implementing the CText Class 807
The CText Constructor 807
Creating a Text Element 807
Drawing a CText Object 809
Moving a CText Object 810
Summary 812
CHAPTER 17: STORING AND PRINTING DOCUMENTS 815
Understanding Serialization 815
Serializing a Document 816
Serialization in the Document Class Defi nition 816
Serialization in the Document Class Implementation 817
The Serialize( ) Function 818
The CArchive Class 818
Functionality of CObject-Based Classes 820
The Macros that Add Serialization to a Class 821
How Serialization Works 821
How to Implement Serialization for a Class 823
Applying Serialization 823
Recording Document Changes 823
Serializing the Document 825
Serializing the Element Classes 827
The Serialize( ) Functions for the Element Classes 829
Exercising Serialization 831
Printing a Document 833
The Printing Process 833
The CPrintInfo Class 835
Implementing Multipage Printing 837
Getting the Overall Document Size 838
Storing Print Data 838
Preparing to Print 839
Cleaning Up after Printing 841
Preparing the Device Context 841
Printing the Document 842
Getting a Printout of the Document 846
Summary 847
CHAPTER 18: PROGRAMMING WINDOWS 8 APPS 849
Understanding Windows 8 Apps 850
Developing Windows 8 Apps 851
Windows Runtime Concepts 852
WinRT Namespaces 852
WinRT Objects 852
C++ Component Extensions (C++/CX) 853
C++/CX Namespaces 853
Defining WinRT Class Types 854
Variables of Ref Class Types 857
Accessing Members of a Ref Class Object 857
Event Handler Functions 858
Casting Ref Class References 858
The eXtensible Application Markup
Language (XAML) 859
XAML Elements 859
UI Elements in XAML 861
Attached Properties 864
Parents and Children 865
Control Elements 865
Layout Elements 865
Handling Events for UI Elements 866
Creating a Windows 8 App 867
Application Files 867
Defining the User Interface 868
Creating the Title 870
Adding Game Controls 872
Creating a Grid to Contain the Cards 874
Defining a Card 875
Creating a Card 875
Adding Event Handling 877
Creating All the Cards 878
Implementing Game Operations 879
Defining the Card Class 880
Adding Data Members to the MainPage Class 881
Adding Function Members 882
Initialize the MainPage Object 883
Initializing the Card Pack 884
Setting Up the Child Elements of cardGrid 884
Initializing the Game 886
Shuffl ing the Cards 889
Highlighting the UI Cards 889
Handling Card Back Events 890
Handling Shape Events 892
Recognizing a Win 894
Handling Game Control Button Events 895
Scaling UI Elements 897
Transitions 899
Application Startup Transitions 899
Storyboard Animations 900
Summary 902
INDEX 905
Ivor Horton is one of the preeminent authors of tutorials on the Java, C, and C++ programming languages. He is widely known for the tutorial style of his books, which provides step-by-step guidance easily understood even by first-time programmers. He is also a systems consultant in private practice.
These books may interest you
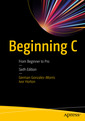
Beginning CFrom Beginner to Pro 68.56 €