Description
C++ Standard Library, The (2nd Ed.)
A Tutorial and Reference
Author: Josuttis Nicolai
Language: English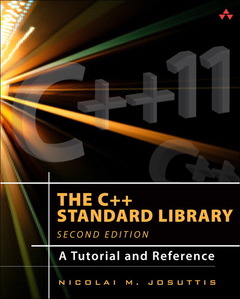
Subject for C++ Standard Library, The:
Approximative price 75.92 €
In Print (Delivery period: 14 days).
Add to cart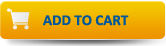
1136 p. · 19x23.8 cm · Hardback
Description
/li>Contents
/li>Biography
/li>Comment
/li>
The Best-Selling C++ Resource
Now Updated for C++11
The C++ standard library provides a set of common classes and interfaces that greatly extend the core C++ language. The library, however, is not self-explanatory. To make full use of its components?and to benefit from their power?you need a resource that does far more than list the classes and their functions.
The C++ Standard Library: A Tutorial and Reference, Second Edition, describes this library as now incorporated into the new ANSI/ISO C++ language standard (C++11). The book provides comprehensive documentation of each library component, including an introduction to its purpose and design; clearly written explanations of complex concepts; the practical programming details needed for effective use; traps and pitfalls; the exact signature and definition of the most important classes and functions; and numerous examples of working code. The book focuses in particular on the Standard Template Library (STL), examining containers, iterators, function objects, and STL algorithms.
The book covers all the new C++11 library components, including
- Concurrency
- Fractional arithmetic
- Clocks and timers
- Tuples
- New STL containers
- New STL algorithms
- New smart pointers
- New locale facets
- Random numbers and distributions
- Type traits and utilities
- Regular expressions
The book also examines the new C++ programming style and its effect on the standard library, including lambdas, range-based for loops, move semantics, and variadic templates.
An accompanying Web site, including source code, can be found at www.cppstdlib.com.
Preface to the Second Edition xxiii
Acknowledgments for the Second Edition xxiv
Preface to the First Edition xxv
Acknowledgments for the First Edition xxvi
Chapter 1: About This Book 1
1.1 Why This Book 1
1.2 Before Reading This Book 2
1.3 Style and Structure of the Book 2
1.4 How to Read This Book 4
1.5 State of the Art 5
1.6 Example Code and Additional Information 5
1.7 Feedback 5
Chapter 2: Introduction to C++ and the Standard Library 7
2.1 History of the C++ Standards 7
2.2 Complexity and Big-O Notation 10
Chapter 3: New Language Features 13
3.1 New C++11 Language Features 13
3.2 Old “New” Language Features 33
Chapter 4: General Concepts 39
4.1 Namespace std 39
4.2 Header Files 40
4.3 Error and Exception Handling 41
4.4 Callable Objects 54
4.5 Concurrency and Multithreading 55
4.6 Allocators 57
Chapter 5: Utilities 59
5.1 Pairs and Tuples 60
5.2 Smart Pointers 76
5.3 Numeric Limits 115
5.4 Type Traits and Type Utilities 122
5.5 Auxiliary Functions 134
5.6 Compile-Time Fractional Arithmetic with Class ratio<> 140
5.7 Clocks and Timers 143
5.8 Header Files <cstddef>, <cstdlib>, and <cstring> 161
Chapter 6: The Standard Template Library 165
6.1 STL Components 165
6.2 Containers 167
6.3 Iterators 188
6.4 Algorithms 199
6.5 Iterator Adapters 210
6.6 User-Defined Generic Functions 216
6.7 Manipulating Algorithms 217
6.8 Functions as Algorithm Arguments 224
6.9 Using Lambdas 229
6.10 Function Objects 233
6.11 Container Elements 244
6.12 Errors and Exceptions inside the STL 245
6.13 Extending the STL 250
Chapter 7: STL Containers 253
7.1 Common Container Abilities and Operations 254
7.2 Arrays 261
7.3 Vectors 270
7.4 Deques 283
7.5 Lists 290
7.6 Forward Lists 300
7.7 Sets and Multisets 314
7.8 Maps and Multimaps 331
7.9 Unordered Containers 355
7.10 Other STL Containers 385
7.11 Implementing Reference Semantics 388
7.12 When to Use Which Container 392
Chapter 8: STL Container Members in Detail 397
8.1 Type Definitions 397
8.2 Create, Copy, and Destroy Operations 400
8.3 Nonmodifying Operations 403
8.4 Assignments 406
8.5 Direct Element Access 408
8.6 Operations to Generate Iterators 410
8.7 Inserting and Removing Elements 411
8.8 Special Member Functions for Lists and Forward Lists 420
8.9 Container Policy Interfaces 427
8.10 Allocator Support 430
Chapter 9: STL Iterators 433
9.1 Header Files for Iterators 433
9.2 Iterator Categories 433
9.3 Auxiliary Iterator Functions 441
9.4 Iterator Adapters 448
9.5 Iterator Traits 466
9.6 Writing User-Defined Iterators 471
Chapter 10: STL Function Objects and Using Lambdas 475
10.1 The Concept of Function Objects 475
10.2 Predefined Function Objects and Binders 486
10.3 Using Lambdas 499
Chapter 11: STL Algorithms 505
11.1 Algorithm Header Files 505
11.2 Algorithm Overview 505
11.3 Auxiliary Functions 517
11.4 The for_each() Algorithm 519
11.5 Nonmodifying Algorithms 524
11.6 Modifying Algorithms 557
11.7 Removing Algorithms 575
11.8 Mutating Algorithms 583
11.9 Sorting Algorithms 596
11.10 Sorted-Range Algorithms 608
11.11 Numeric Algorithms 623
Chapter 12: Special Containers 631
12.1 Stacks 632
12.2 Queues 638
12.3 Priority Queues 641
12.4 Container Adapters in Detail 645
12.5 Bitsets 650
Chapter 13: Strings 655
13.1 Purpose of the String Classes 656
13.2 Description of the String Classes 663
13.3 String Class in Detail 693
Chapter 14: Regular Expressions 717
14.1 The Regex Match and Search Interface 717
14.2 Dealing with Subexpressions 720
14.3 Regex Iterators 726
14.4 Regex Token Iterators 727
14.5 Replacing Regular Expressions 730
14.6 Regex Flags 732
14.7 Regex Exceptions 735
14.8 The Regex ECMA Script Grammar 738
14.9 Other Grammars 739
14.10 Basic Regex Signatures in Detail 740
Chapter 15: Input/Output Using Stream Classes 743
15.1 Common Background of I/O Streams 744
15.2 Fundamental Stream Classes and Objects 748
15.3 Standard Stream Operators << and >> 753
15.4 State of Streams 758
15.5 Standard Input/Output Functions 767
15.6 Manipulators 774
15.7 Formatting 779
15.8 Internationalization 790
15.9 File Access 791
15.10 Stream Classes for Strings 802
15.11 Input/Output Operators for User-Defined Types 810
15.12 Connecting Input and Output Streams 819
15.13 The Stream Buffer Classes 826
15.14 Performance Issues 844
Chapter 16: Internationalization 849
16.1 Character Encodings and Character Sets 850
16.2 The Concept of Locales 857
16.3 Locales in Detail 866
16.4 Facets in Detail 869
Chapter 17: Numerics 907
17.1 Random Numbers and Distributions 907
17.2 Complex Numbers 925
17.3 Global Numeric Functions 941
17.4 Valarrays 943
Chapter 18: Concurrency 945
18.1 The High-Level Interface: async() and Futures 946
18.2 The Low-Level Interface: Threads and Promises 964
18.3 Starting a Thread in Detail 973
18.4 Synchronizing Threads, or the Problem of Concurrency 982
18.5 Mutexes and Locks 989
18.6 Condition Variables 1003
18.7 Atomics 1012
Chapter 19: Allocators 1023
19.1 Using Allocators as an Application Programmer 1023
19.2 A User-Defined Allocator 1024
19.3 Using Allocators as a Library Programmer 1026
Bibliography 1031
Index 1037
Nicolai M. Josuttis is an independent technical consultant who designs mid-sized and large software systems for the telecommunication, traffic, finance, and manufacturing industries. A former member of the C++ Standard Committee library working group, he is well known in the programming community for his authoritative books. In addition to The C++ Standard Library, a worldwide best-seller since its first publication in 1999, his books include C++ Templates: The Complete Guide (with David Vandevoorde, Addison-Wesley, 2003) and SOA in Practice: The Art of Distributed System Design (O’Reilly Media, 2007).
- Thoroughly documents each library component incorporated in the brand-new C++ standard
- Clearly explains complex concepts, and presents the practical detail programmers need to use the Standard Library effectively
- Contains many examples of working code, all available for download at an accompanying website
These books may interest you
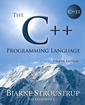
C++ Programming Language, The 80.31 €
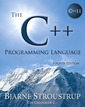
C++ Programming Language, The 75.92 €